게임은 정말 정식출시를 하더라도 끝이 아니라는것을 깨달았다.
끊임없이 피드백이 들어오고 불편한점, 요구사항들이 몰려온다.
물론 너무 사람들의 입맛에만 맞춰 줏대없이 흔들리는건 게임의 정체성을 희미하게 만들수 있겠지만
그래도 사용자들의 피드백을 잘 듣고 추가나 제거가 필요한 부분들은 수정이 필요하다
1. 초반부터 난이도가 너무 어려움
2. 공을 발사시 발사 방향을 알려줬으면 좋겠음
지금까지 주로 많은 사람들에게 받은 피드백이자, 나 역시 수정하는게 맞다고 결정한 부분들이다
우선 초반부터 난이도가 너무 어렵다고 하니 밸런스 조절을 해주자
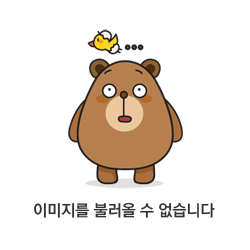
우선 극초반 스테이지에 나오는 유닛들은 기본 유닛이라도 HP를 더 줄여주고
발사시간은 대폭 늘려주었다
생각해보니 초반 스테이지는 훨씬 더 쉽게 해주고 기본 유닛 스탯 자체를 좀 더 쉽게 해줄 필요가 있다 생각했다
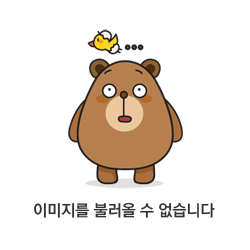
특히 발사시간! 최소 3초에서 5초 사이에 한번씩 발사하면 게임창이 순식간에 적의 공으로 가득 차게된다
나처럼 이미 고인물들이라면 모를까 처음 해보는 사람 입장에서는 너무 어려울것 같아 고쳐주었다
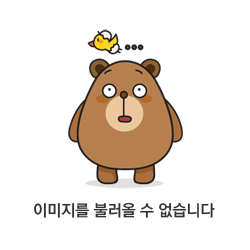
특히 얘는 3~5초에 한번씩 발사하게 했다니... 내가 정말 밸런스를 생각 안하긴 했었다
2. 공을 발사시 발사 방향을 알려줬으면 좋겠음
두번째 피드백인 발사 방향을 알려주기! 한번 바로 구현해보자
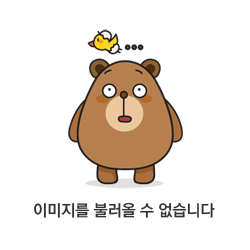
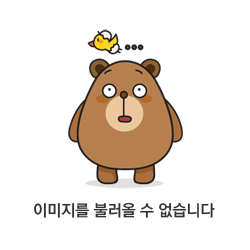
우선 화살표 무료 이미지를 받아와 배경을 제거해주자
애초에 원본을 위로 향하는 방향이 아닌 왼쪽을 향하도록 한 이유는
유니티에서 2D 스프라이트나 UI 오브젝트는 기본적으로 오른쪽(→)이 정방향 이라고 한다
그렇기에 처음부터 왼쪽을 향하는 이미지로 프리팹을 만들어야지 공과 함께 생성시 앞을 바라보게 된다
using System;
using UnityEngine;
using UnityEngine.SceneManagement;
using TMPro;
public class SPGameManager : MonoBehaviour
{
public GameObject point; // 추가된 point 오브젝트
private GameObject currentPoint; // 현재 생성된 point 오브젝트
private void Update()
{
if (Input.GetMouseButtonDown(0))
{
clickPosition = Camera.main.ScreenToWorldPoint(Input.mousePosition);
clickPosition.z = 0f;
Collider2D[] colliders = Physics2D.OverlapPointAll(clickPosition);
foreach (Collider2D collider in colliders)
{
if (collider.gameObject == P1firezone)
{
GameObject spawnedObject;
if (fireitem != null)
{
spawnedObject = Instantiate(fireitem, clickPosition, Quaternion.identity);
Debug.Log("P1이 아이템을 사용하였습니다");
Debug.Log("아이템의 이름은 " + fireitem.gameObject.name + "입니다");
fireitem = null;
}
else
{
spawnedObject = Instantiate(P1ballPrefab, clickPosition, Quaternion.identity);
Debug.Log("P1이 기본구체를 날렸습니다");
}
currentPoint = Instantiate(point, spawnedObject.transform.position + new Vector3(0, 0.65f, 0), Quaternion.identity);
AddBall();
isDragging = true;
break;
}
}
}
if (isDragging && Input.GetMouseButtonUp(0))
{
Vector3 currentPosition = Camera.main.ScreenToWorldPoint(Input.mousePosition);
currentPosition.z = 0f;
shotDistance = Vector3.Distance(clickPosition, currentPosition) * 2;
Vector3 dragDirection = (currentPosition - clickPosition).normalized;
shotDirection = dragDirection;
isDragging = false;
if (currentPoint != null)
{
Destroy(currentPoint); // 마우스 클릭을 떼는 순간 point 오브젝트 제거
}
}
}
....
}
이제 공이나 아이템이 생성될때마다 y축으로 0.65만큼 위에서 화살표도 함께 생성된다.
물론 클릭(터치) 상태일때만 생성되고 클릭(터치)가 떼지는 순간 화살표는 제거된다
영상에서 공 발사마다 화살표가 생성되고 클릭을 떼는순간 화살표가 제거되는 것을 확인할 수 있다
이제 화살표 생성까지는 성공적으로 됐으니 이번엔 마우스의 방향에 따라 실시간으로
화살표가 움직이도록 해보자
private void Update()
{
if (Input.GetMouseButtonDown(0))
{
clickPosition = Camera.main.ScreenToWorldPoint(Input.mousePosition);
clickPosition.z = 0f;
Collider2D[] colliders = Physics2D.OverlapPointAll(clickPosition);
foreach (Collider2D collider in colliders)
{
if (collider.gameObject == P1firezone)
{
GameObject spawnedObject;
if (fireitem != null)
{
spawnedObject = Instantiate(fireitem, clickPosition, Quaternion.identity);
Debug.Log("P1이 아이템을 사용하였습니다");
Debug.Log("아이템의 이름은 " + fireitem.gameObject.name + "입니다");
fireitem = null;
}
else
{
spawnedObject = Instantiate(P1ballPrefab, clickPosition, Quaternion.identity);
Debug.Log("P1이 기본구체를 날렸습니다");
}
currentPoint = Instantiate(point, spawnedObject.transform.position + new Vector3(0, 0.65f, 0), Quaternion.Euler(0, 0, 90));
AddBall();
isDragging = true;
break;
}
}
}
if (isDragging)
{
Vector3 currentMousePosition = Camera.main.ScreenToWorldPoint(Input.mousePosition);
currentMousePosition.z = 0f;
if (currentPoint != null)
{
Vector3 direction = (currentPoint.transform.position - currentMousePosition).normalized; // 방향 반전
float angle = Mathf.Atan2(direction.y, direction.x) * Mathf.Rad2Deg;
currentPoint.transform.rotation = Quaternion.Euler(0, 0, angle);
}
}
if (isDragging && Input.GetMouseButtonUp(0))
{
Vector3 currentPosition = Camera.main.ScreenToWorldPoint(Input.mousePosition);
currentPosition.z = 0f;
shotDistance = Vector3.Distance(clickPosition, currentPosition) * 2;
Vector3 dragDirection = (currentPosition - clickPosition).normalized;
shotDirection = dragDirection;
isDragging = false;
if (currentPoint != null)
{
Destroy(currentPoint); // 마우스 클릭을 떼는 순간 point 오브젝트 제거
}
}
}
당연히 실시간으로 화살표가 움직여야 하니 update문에 추가해주었고
마우스 포지션 위치를 Vector3 타입 변수 currentPosition에 받아와 움직이도록 해주었다
이제 제법 그럴싸하게 구현되는것을 확인할 수 있다
'Galaxy Ball > 6. 기타 수정 + 추가 기능' 카테고리의 다른 글
영어 기능 지원#2 + 기타 업데이트 (0) | 2025.01.14 |
---|---|
영어 기능 지원#1 (0) | 2025.01.10 |
자잘한 수정 + 게임 이쁘게 다듬기 (1) | 2025.01.05 |